ANP去中心化身份实现指南 | DID技术细节与最佳实践
ANP去中心化身份实现指南 | DID技术细节与最佳实践
去中心化身份实现指南
本文档提供ANP中去中心化身份(DID)的详细实现指南,涵盖从基础概念到实际代码的全面指引,帮助开发者理解和实现ANP的身份与加密通信层。
DID基础概念
去中心化身份标识符(DID)是ANP身份层的核心组件,它具有以下关键特性:
- 全球唯一性:每个DID在全球范围内唯一标识一个智能体
- 去中心化控制:身份控制者无需依赖中央机构
- 加密验证:通过密码学方式验证身份
- 永久性:DID一旦创建可以永久存在
- 可解析性:DID可解析获取相关身份信息
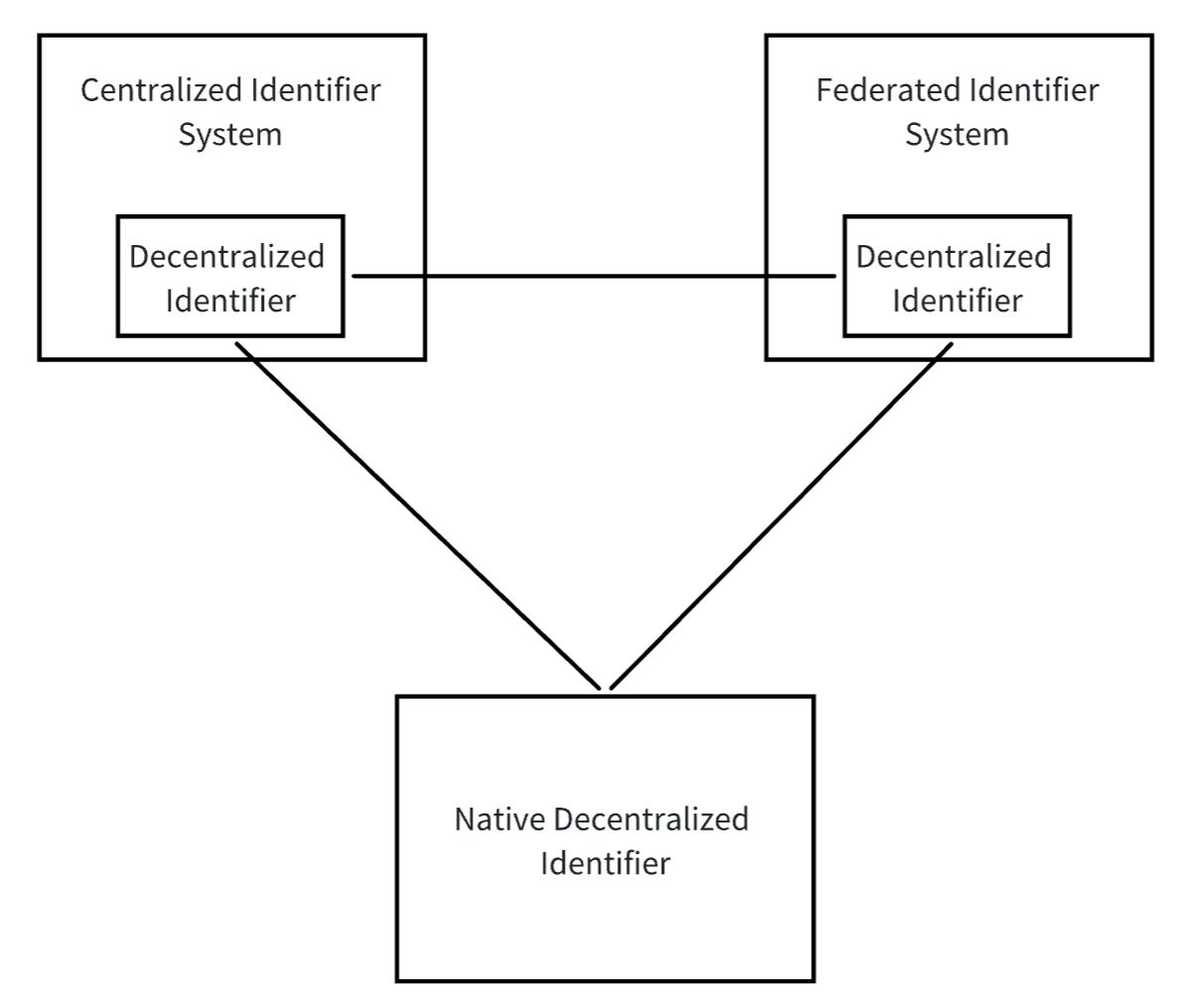
图1:DID作为连接不同身份系统的互操作桥梁
DID格式与组成
ANP支持标准的DID格式:
did:anp:123456789abcdefghi
| | |
| | └── 特定标识符(方法特定)
| └── 方法名称(anp)
└── DID前缀
ANP默认支持以下DID方法:
did:anp:
:ANP原生DID方法did:web:
:基于Web的DID方法did:key:
:基于公钥的简化DID方法
DID文档结构
DID文档是描述DID控制者各种属性的JSON-LD文档:
{
"@context": [
"https://www.w3.org/ns/did/v1",
"https://anp.org/ns/did/v1"
],
"id": "did:anp:123456789abcdefghi",
"controller": "did:anp:123456789abcdefghi",
"verificationMethod": [
{
"id": "did:anp:123456789abcdefghi#keys-1",
"type": "Ed25519VerificationKey2020",
"controller": "did:anp:123456789abcdefghi",
"publicKeyMultibase": "zH3C2AVvLMv6gmMNam3uVAjZpfkcJCwDwnZn6z3wXmqPV"
}
],
"authentication": [
"did:anp:123456789abcdefghi#keys-1"
],
"assertionMethod": [
"did:anp:123456789abcdefghi#keys-1"
],
"keyAgreement": [
{
"id": "did:anp:123456789abcdefghi#keys-2",
"type": "X25519KeyAgreementKey2020",
"controller": "did:anp:123456789abcdefghi",
"publicKeyMultibase": "z6LSbgq3GejX88eiAYbYAP2nQD8XvjF9VYR3LYnPNNN4V"
}
],
"service": [
{
"id": "did:anp:123456789abcdefghi#messaging",
"type": "ANPMessagingService",
"serviceEndpoint": "https://messaging.example.com/agent/123456789abcdefghi"
},
{
"id": "did:anp:123456789abcdefghi#profile",
"type": "AgentProfile",
"serviceEndpoint": "https://profiles.example.com/agents/123456789abcdefghi"
}
],
"created": "2024-03-20T14:15:22Z",
"updated": "2024-03-20T14:15:22Z"
}
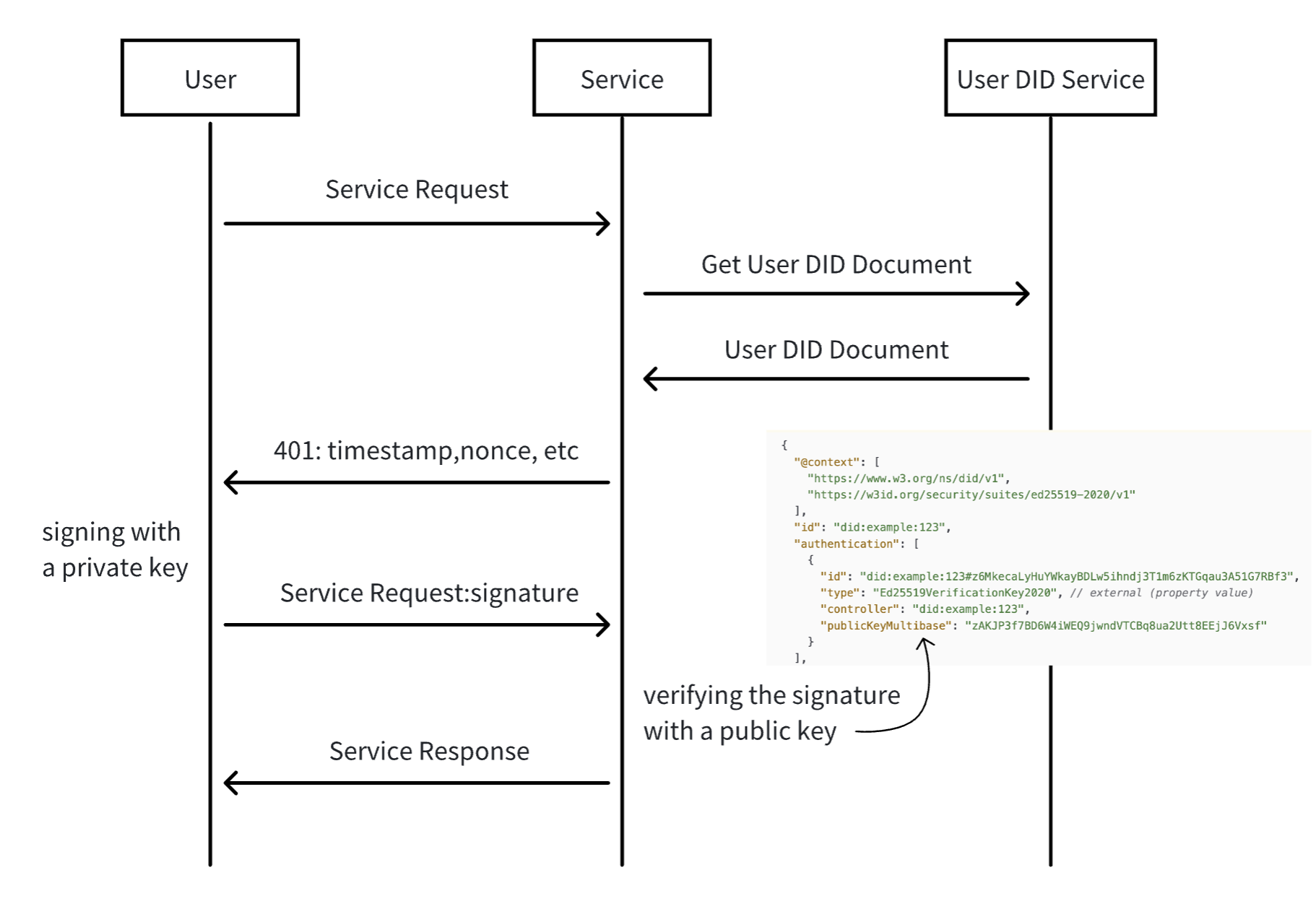
图2:DID文档结构与身份验证流程
DID生命周期管理
DID创建
创建DID的完整流程:
- 生成密钥对:创建用于身份验证的非对称密钥对
- 构造DID标识符:基于密钥或随机值生成唯一标识符
- 构建DID文档:创建包含公钥和相关信息的文档
- 注册DID:根据选择的DID方法注册DID(如适用)
示例代码(TypeScript)
import { generateKeyPair, randomBytes } from 'crypto';
import { encode } from 'multibase';
// 生成Ed25519密钥对
async function generateEd25519KeyPair() {
return new Promise((resolve, reject) => {
generateKeyPair('ed25519', {
publicKeyEncoding: { type: 'spki', format: 'pem' },
privateKeyEncoding: { type: 'pkcs8', format: 'pem' }
}, (err, publicKey, privateKey) => {
if (err) reject(err);
resolve({ publicKey, privateKey });
});
});
}
// 创建ANP DID
async function createAnpDID() {
// 1. 生成密钥对
const { publicKey, privateKey } = await generateEd25519KeyPair();
// 2. 生成唯一标识符
const idBytes = randomBytes(16);
const identifier = encode('base58btc', idBytes).slice(1); // 去除前缀
// 3. 格式化DID标识符
const did = `did:anp:${identifier}`;
// 4. 格式化公钥为multibase
const publicKeyBytes = Buffer.from(publicKey);
const publicKeyMultibase = encode('base58btc', publicKeyBytes).slice(1);
// 5. 构造DID文档
const didDocument = {
"@context": [
"https://www.w3.org/ns/did/v1",
"https://anp.org/ns/did/v1"
],
"id": did,
"controller": did,
"verificationMethod": [
{
"id": `${did}#keys-1`,
"type": "Ed25519VerificationKey2020",
"controller": did,
"publicKeyMultibase": publicKeyMultibase
}
],
"authentication": [ `${did}#keys-1` ],
"assertionMethod": [ `${did}#keys-1` ],
"service": [
{
"id": `${did}#messaging`,
"type": "ANPMessagingService",
"serviceEndpoint": `https://example.com/agents/${identifier}`
}
],
"created": new Date().toISOString(),
"updated": new Date().toISOString()
};
return {
did,
didDocument,
privateKey
};
}
DID解析
解析DID获取其文档的过程:
- 解析DID方法:确定DID使用的方法
- 调用方法解析器:根据方法执行特定的解析逻辑
- 验证DID文档:确保文档符合标准且有效
- 返回DID文档:返回验证后的DID文档
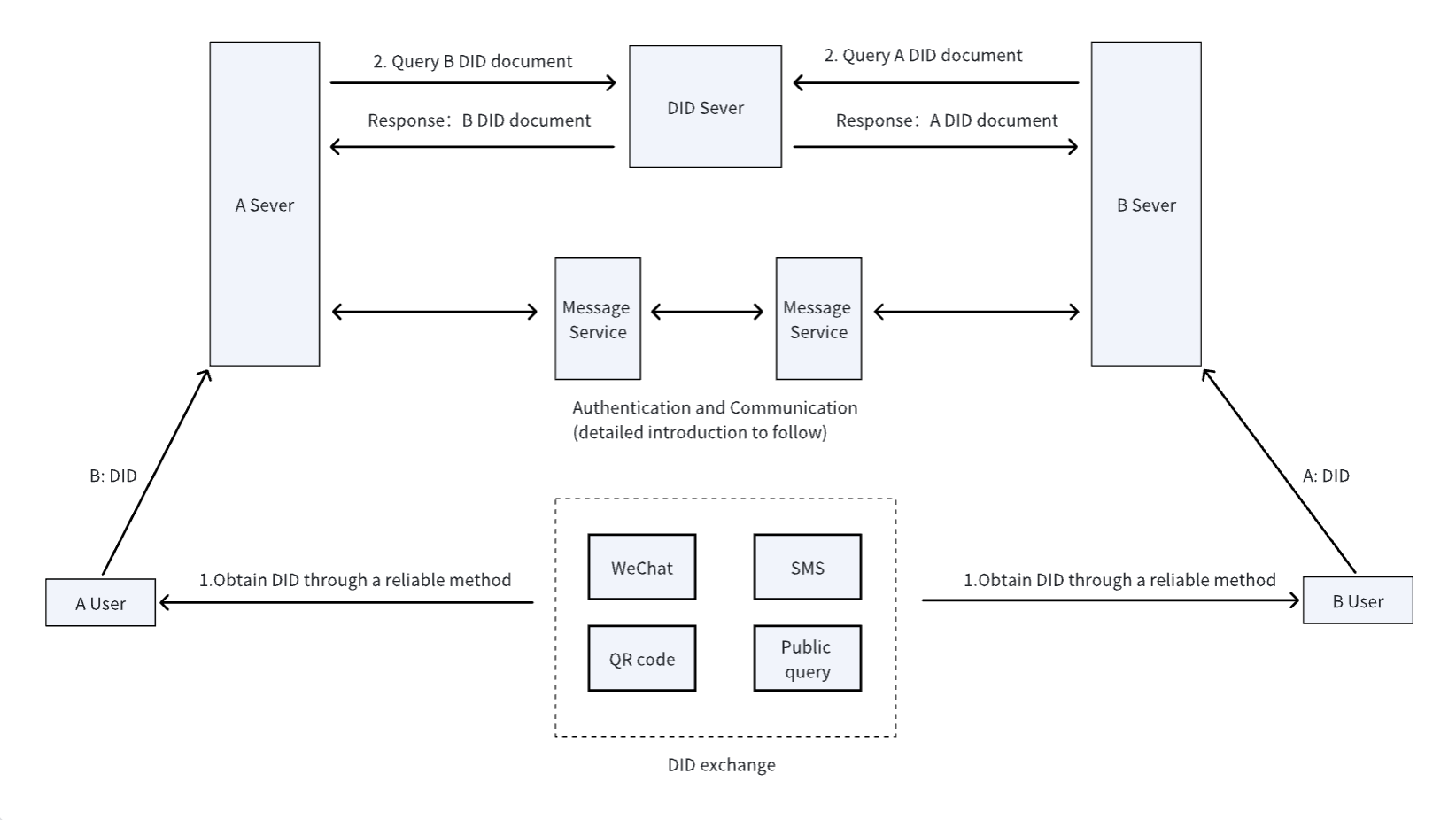
图3:基于DID的跨平台身份认证与解析流程
示例代码(TypeScript)
// DID解析器类
class DIDResolver {
private methodResolvers = new Map<string, MethodResolver>();
// 注册方法解析器
registerMethod(method: string, resolver: MethodResolver) {
this.methodResolvers.set(method, resolver);
}
// 解析DID
async resolve(did: string): Promise<DIDDocument | null> {
try {
// 解析DID格式
const [prefix, method, identifier] = did.split(':');
if (prefix !== 'did') {
throw new Error('Invalid DID format: missing "did" prefix');
}
// 获取方法解析器
const resolver = this.methodResolvers.get(method);
if (!resolver) {
throw new Error(`Unsupported DID method: ${method}`);
}
// 执行方法特定的解析
const didDocument = await resolver.resolve(identifier);
// 验证DID文档
if (!this.validateDIDDocument(didDocument, did)) {
throw new Error('Invalid DID Document');
}
return didDocument;
} catch (error) {
console.error('DID resolution error:', error);
return null;
}
}
// 验证DID文档
private validateDIDDocument(document: DIDDocument, did: string): boolean {
// 实现文档验证逻辑
return document.id === did &&
document['@context'].includes('https://www.w3.org/ns/did/v1') &&
document.verificationMethod &&
document.verificationMethod.length > 0;
}
}
// 接口定义
interface MethodResolver {
resolve(identifier: string): Promise<DIDDocument>;
}
interface DIDDocument {
'@context': string[];
id: string;
controller: string;
verificationMethod: any[];
authentication: any[];
[key: string]: any;
}
DID验证
验证DID身份真实性的过程:
- 解析DID文档:获取与DID关联的文档
- 提取验证方法:找到相关的验证方法和公钥
- 验证签名:使用公钥验证数字签名
- 检查有效性:确认DID和相关证明的有效性
密钥管理
ANP身份实现中的密钥管理最佳实践:
密钥类型
ANP推荐的密钥类型:
- Ed25519:用于数字签名,性能高、安全性强
- X25519:用于密钥协商和加密
- secp256k1:兼容性较好,适用于特定场景
密钥存储安全
- 安全存储:使用加密的密钥存储
- 访问控制:严格限制密钥访问权限
- 内存保护:最小化密钥在内存中的暴露时间
- 硬件保护:条件允许时使用硬件安全模块(HSM)
密钥轮换
- 定期轮换:定期更新密钥增强安全性
- 轮换流程:
- 生成新密钥对
- 使用旧密钥签署新公钥
- 更新DID文档添加新验证方法
- 将新密钥标记为首选
- 保留旧密钥一段时间后安全删除
高级身份功能
可验证凭证
ANP支持与DID结合的可验证凭证(VC):
{
"@context": [
"https://www.w3.org/2018/credentials/v1",
"https://anp.org/schemas/credentials/v1"
],
"id": "http://example.com/credentials/1234",
"type": ["VerifiableCredential", "AgentCapabilityCredential"],
"issuer": "did:anp:issuer123456789",
"issuanceDate": "2024-03-20T12:00:00Z",
"credentialSubject": {
"id": "did:anp:123456789abcdefghi",
"capabilities": [
{
"type": "DataProcessing",
"level": "Advanced",
"description": "能够处理和分析复杂数据集"
}
]
},
"proof": {
"type": "Ed25519Signature2020",
"created": "2024-03-20T12:05:00Z",
"verificationMethod": "did:anp:issuer123456789#keys-1",
"proofPurpose": "assertionMethod",
"proofValue": "z3aq9h5bgsjjbJMzDtEEBKnCfgFJR6KkYpTPRUEKoWCvwQhzjBj9Rz2o4YcdQr9BEJ8sGF..."
}
}
授权委托
ANP支持智能体间的授权委托机制:
- 委托创建:委托者创建授权委托文档
- 委托签名:委托者对文档进行签名
- 委托验证:验证者核实委托有效性
- 权限执行:按照委托内容执行授权操作
互操作性考量
ANP身份实现与其他系统的互操作:
- SSI生态系统:与自主身份(SSI)生态系统兼容
- 其他DID方法:支持常见DID方法的互操作
- VC标准:兼容W3C可验证凭证标准
- 身份钱包:支持与标准身份钱包集成
常见问题与解决方案
处理DID解析失败
- 多重解析:尝试备用解析器或方法
- 缓存机制:缓存已解析的DID文档
- 优雅降级:设计在解析失败时的降级策略
安全防护
- 防重放攻击:使用nonce和时间戳
- 防篡改:验证所有消息的完整性
- 防钓鱼:验证DID和公钥的一致性
实现路线图
ANP身份实现的建议开发路线:
- 基础实现:实现DID创建、解析和基本验证
- 加密通信:添加基于DID的端到端加密
- 凭证系统:实现可验证凭证支持
- 高级功能:添加委托授权、多设备支持等
- 优化集成:提高性能和各系统集成能力
通过遵循这些详细指南,开发者可以实现符合ANP标准的去中心化身份系统,为智能体提供安全、可靠的身份基础。